Example program 2: MM_S2_Viz_Basic
Program Introduction
Description |
The robot triggers the Mech-Viz project to run, and then obtains the planned path for picking and placing. |
File path |
You can navigate to the installation directory of Mech-Vision and Mech-Viz and find the file by using the |
Project |
Mech-Vision and Mech-Viz projects |
Prerequisites |
|
This example program is provided for reference only. Before using the program, please modify the program according to the actual scenario. |
Program Description
This part describes the MM_S2_Viz_Basic example program.
DEF MM_S2_Viz_Basic ( )
;---------------------------------------------------
; FUNCTION: trigger Mech-Viz project and get
; planned path
; Mech-Mind, 2023-12-25
;---------------------------------------------------
;set current tool no. to 1
BAS(#TOOL,1)
;set current base no. to 0
BAS(#BASE,0)
;move to robot home position
PTP HOME Vel=100 % DEFAULT
;initialize communication parameters (initialization is required only once)
MM_Init_Socket("XML_Kuka_MMIND",873,871,60)
;move to image-capturing position
LIN camera_capture Vel=1 m/s CPDAT1 Tool[1] Base[0]
;trigger Mech-Viz project
MM_Start_Viz(2,init_jps)
;get planned path, 1st argument (1) means getting pose in JPs
MM_Get_VizData(1,pos_num,vis_pos_num,status)
;check whether planned path has been got from Mech-Viz successfully
IF status<> 2100 THEN
;add error handling logic here according to different error codes
;e.g.: status=2038 means no point cloud in ROI
halt
ENDIF
;save waypoints of the planned path to local variables one by one
MM_Get_Jps(1,Xpick_point1,label[1],toolid[1])
MM_Get_Jps(2,Xpick_point2,label[2],toolid[2])
MM_Get_Jps(3,Xpick_point3,label[3],toolid[3])
;follow the planned path to pick
;move to approach waypoint of picking
PTP pick_point1 Vel=50 % PDAT1 Tool[1] Base[0]
;move to picking waypoint
PTP pick_point2 Vel=10 % PDAT2 Tool[1] Base[0]
;add object grasping logic here, such as "$OUT[1]=TRUE"
halt
;move to departure waypoint of picking
PTP pick_point3 Vel=50 % PDAT3 Tool[1] Base[0]
;move to intermediate waypoint of placing
PTP drop_waypoint CONT Vel=100 % PDAT2 Tool[1] Base[0]
;move to approach waypoint of placing
LIN drop_app Vel=1 m/s CPDAT3 Tool[1] Base[0]
;move to placing waypoint
LIN drop Vel=0.3 m/s CPDAT4 Tool[1] Base[0]
;add object releasing logic here, such as "$OUT[1]=FALSE"
halt
;move to departure waypoint of placing
LIN drop_app Vel=1 m/s CPDAT3 Tool[1] Base[0]
;move back to robot home position
PTP HOME Vel=100 % DEFAULT
END
The workflow corresponding to the above example program code is shown in the figure below.
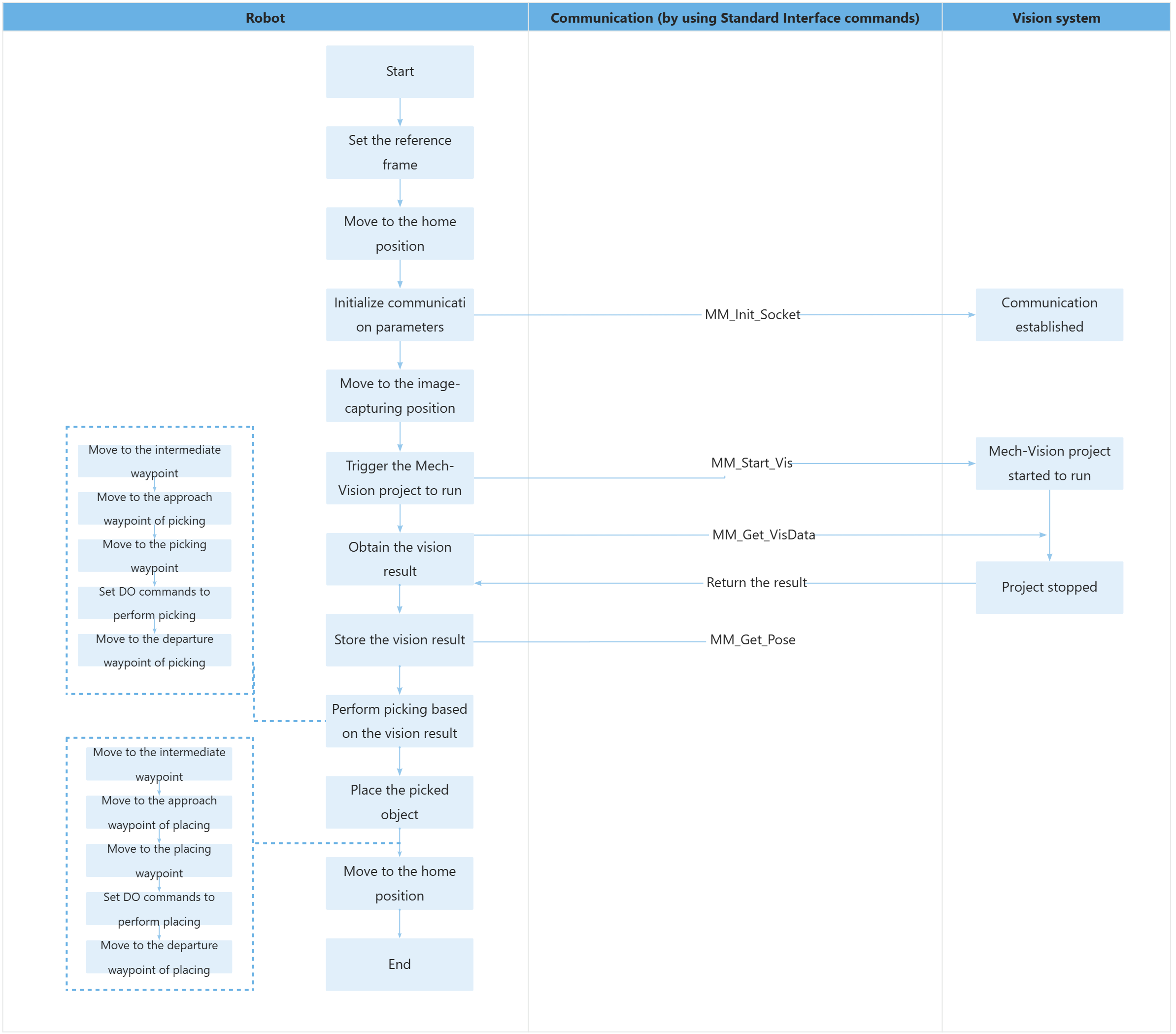
The table below explains the above program. You can click the hyperlink to the command name to view its detailed description.
Feature | Code and description | ||
---|---|---|---|
Set the reference frame |
The above two statements set the current tool and base reference frames. |
||
Move to the home position |
The above statement specifies to move the robot in PTP mode to the taught home position. |
||
Initialize communication parameters |
The MM_Init_Socket command establishes the TCP communication between the robot and the vision system based on the configurations in the XML_Kuka_MMIND.xml file.
|
||
Move to the image-capturing position |
The above statement specifies to move the robot linearly to the taught image-capturing position. |
||
Trigger the Mech-Viz project to run |
The entire command indicates that the robot triggers the vision system to run the Mech-Viz project, and then Mech-Viz plans the robot’s picking path base on the vision result output by Mech-Vision. |
||
Get the planned path |
The entire statement indicates that the robot obtains the planned path from the Mech-Viz project.
The above statement indicates that when the status code is 2100, the robot has successfully obtained the planned path; otherwise, an exception has occurred in the vision system and the program executes the code between IF and ENDIF. You can perform the corresponding operation based on the specific error code. In this example program, all error codes are handled in the same way, by pausing the program execution using the halt command. |
||
Store the planned path |
The entire statement “MM_Get_Jps(1,Xpick_point1,label[1],toolid[1])” stores the joint positions, label, and tool ID of the first waypoint in the specified variables.
|
||
Move to the approach waypoint of picking |
The robot moves to the approach waypoint of picking. pick_point1 and Xpick_point1 indicate the same position. |
||
Move to the picking waypoint |
The robot moves to the picking waypoint. pick_point2 and Xpick_point2 indicate the same position. |
||
Set DOs to perform picking |
After the robot moves to the picking waypoint, you can set a DO (such as “$OUT[1]=TRUE”) to control the robot to use the tool to perform picking. Please set DOs based on the actual situation.
|
||
Move to the departure waypoint of picking |
The robot moves to the departure waypoint of picking. pick_point3 and Xpick_point3 indicate the same position. |
||
Move to the intermediate waypoint |
The robot moves to a intermediate waypoint (drop_waypoint) between the departure waypoint of picking and the approach waypoint of placing.
|
||
Move the robot to the approach waypoint of placing |
The robot moves from the intermediate waypoint to the approach waypoint of placing (drop_app).
|
||
Move to the placing waypoint |
The robot moves from the approach waypoint of placing to the placing waypoint (drop).
|
||
Set DOs to perform placing |
After the robot moves to the placing waypoint, you can set a DO (such as “$OUT[1]=FALSE”) to control the robot to use the tool to perform placing. Please set DOs based on the actual situation.
|
||
Move the robot to the departure waypoint of placing |
The robot moves from the placing waypoint to the departure waypoint of placing (drop_app).
|
||
Move to the home position |
The robot moves from the departure waypoint of placing to the home waypoint again.
|