Kawasaki Standard Interface Functions
When writing your own program, pay attention to the following:
-
Multiple parameters in a function should be separated by commas.
-
Variable-type parameters should use local variables, which take effect only in the program.
-
Input or Output parameters can be customized for functions.
-
Arguments in the program: the input arguments can be constants, global variables or local variables, and the output arguments can be global variables or local variables.
Initialize Communication
Start Mech-Vision Project
This function starts the Mech-Vision project that executes image capturing and performs vision recognition. It applies for applications that use only Mech-Vision but not Mech-Viz.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
.pos_num_need |
The expected number of vision points for Mech-Vision to send. Value range: 0 to 20, in which 0 indicates that all vision points will be sent. |
.sendpos_type |
The type of the robot pose to be sent to Mech-Vision. Value range: 0 to 3.
|
.#start_vis |
The robot joint positions of the start point when .sendpos_type is set to 3. When .sendpos_type is set other values, this parameter can be directly set to #start_vis. |
Example
-
Example 1:
CALL mm_start_vis(1,1,1,#start_vis)
defaultThis example triggers the Mech-Vision project whose ID is 1 to run; the Mech-Vision project is expected to send back one vision point; the robot sends its current joint positions and flange pose as the image-capturing pose to Mech-Vision. The #start_vis parameter is not specified.
-
Example 2:
CALL mm_start_vis(1,1,3,#start_vis)
defaultThis example triggers the Mech-Vision project whose ID is 1 to run; the Mech-Vision project is expected to send back one vision point; The robot sends the joint positions stored in the #start_vis variable to Mech-Vision as the start point of the planned path for the simulated robot in the Path Planning tool.
Get Vision Target(s)
This function obtains the vision result from the corresponding Mech-Vision project. It applies for applications that use Mech-Vision but not Mech-Viz.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of returned vision points. |
.ret |
Name of the variable for storing the status code. Please see Status Codes and Troubleshooting for detailed information. |
Start Mech-Viz Project
This function starts the Mech-Viz project that triggers the corresponding Mech-Vision project, and set the initial pose of the simulated robot in Mech-Viz. It applies for applications that use both Mech-Vision and Mech-Viz.
Parameter
Input parameter | Description |
---|---|
.sendpos_type |
Robot pose type. Value range: 0 to 2.
|
.#start_viz |
The robot joint positions of the start point when .sendpos_type is set to 2. When .sendpos_type is set other values, this parameter can be directly set to #start_viz. |
Example
-
Example 1:
CALL mm_start_viz(1,#start_viz)
defaultThis function triggers the Mech-Viz project to run and sends the current joint positions and flange pose of the robot to Mech-Viz.
-
Example 2:
CALL mm_start_viz(2,#start_viz)
defaultThis function triggers the Mech-Viz project to run and sends the joint positions stored in #start_viz to Mech-Viz.
Get Planned Path from Mech-Viz
This function obtains the path planned by a Mech-Viz project.
Parameter
Input parameter | Description |
---|---|
.getpos_type |
Pose type of the waypoints on the planned path sent by Mech-Viz. Value range: 1 or 2.
|
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received waypoints. |
.vispos_num |
Name of the variable for storing the position of the first Vision Move waypoint in the path. For example, if the workflow is: Fixed-Point Move 1 → Fixed-Point Move 2 → Vision Move → Fixed-Point Move 3, the value of VisPose_Num (the position of the Vision Move Step) is 3. If there is no Vision Move Step in the workflow, the parameter value is 0. |
.ret |
Name of the variable for storing the status code. Please see Status Codes and Troubleshooting for detailed information. |
Example
CALL mm_get_vizdata(2,posnum,vis_index,statuscode)
This example obtains the planned path from Mech-Viz in the form of TCPs. The number of waypoints received is stored in variable posnum, the position of the Vision Move waypoint is stored in variable vis_index, and the received status code is stored in variable statuscode.
Store an Obtained TCP Pose
This function stores the TCP pose of a vision point returned by Mech-Vision or a waypoint returned by Mech-Vision or Mech-Viz in the specified variable.
Parameter
Input parameter | Description |
---|---|
.index |
The index of the vision point or waypoint whose TCP pose will be stored. |
Output parameter | Description |
---|---|
&targetpos |
The name of the pose variable for storing the specified TCP pose. The “&” needs to be put in front of the parameter to indicate that the value of this variable is a TCP pose. |
.label |
Name of the variable for storing the label corresponding to the specified TCP pose. |
.speed |
Name of the variable for storing the velocity corresponding to the specified TCP pose. |
Store the Joint Positions of an Obtained Waypoint
This function stores the joint positions of a waypoint on the planned path returned by Mech-Vision or Mech-Viz in the specified variable.
Parameter
Input parameter | Description |
---|---|
.index |
The index of the vision point or waypoint whose TCP pose will be stored. |
Output parameter | Description |
---|---|
.#targetpos |
Name of the JPs variable for storing a specified set of joint positions. The “#” needs to be put in front of the parameter to indicate that the value of this variable is a set of joint positions. |
.label |
Name of the variable for storing the label corresponding to the specified TCP pose. |
.speed |
Name of the variable for storing the velocity corresponding to the specified TCP pose. |
Switch Mech-Vision Recipe
This function specifies which parameter recipe of the Mech-Vision project to use. This function must be called BEFORE “mm_start_vis”.
Select Mech-Viz Branch
This function selects along which branch the Mech-Viz project should proceed. Such branching is achieved by adding “Branch by Msg” Step(s) to the Mech-Viz project. This function specifies which exit port such Step(s) should take. “mm_start_viz” must be called BEFORE this function. When the Mech-Viz project executes to the “Branch by Msg” Step, it will wait for “mm_set_branch” to send the exit port of the branch.
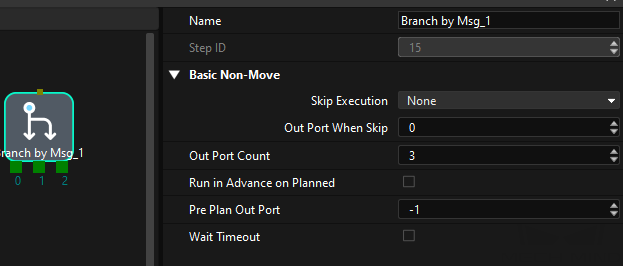
Parameter
Input parameter | Description |
---|---|
.branch_num |
Step ID of the “Branch by Msg” Step. Its value is an integer. The Step ID is displayed in the parameters of the Step. |
.exit_num |
The number of the exit port to take + 1. For example, to select exit port 0 in Mech-Viz, set the value of this parameter to 1. Value range: 1 to 99. |
Set Move Index
This command sets the value for the Current Index parameter of a Mech-Viz Step. Steps that have this parameter include Move by List, Move by Grid, Custom Pallet Pattern, and Predefined Pallet Pattern. “mm_start_viz” must be called BEFORE this function.
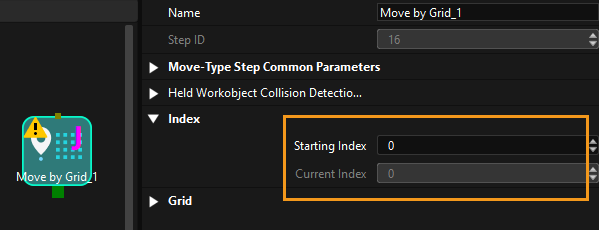
Parameter
Input parameter | Description |
---|---|
.skill_num |
ID of the Step, an integer. The Step ID is displayed in the parameters of the Step. |
.index_num |
The index value that should be set the next time this Step is executed. When this function is called, the current index value in Mech-Viz will become the parameter value minus 1. When the Mech-Viz project runs to the Step specified by this function, the Current Index value in Mech-Viz will be increased by 1 to become the parameter’s value. |
Get Software Status
This function is used to obtain the software execution status of Mech-Vision, Mech-Viz, and Mech-Center. Currently, this function can only be used to check whether Mech-Vision is ready to run.
Parameter
Output parameter | Description |
---|---|
.ret |
Name of the variable for storing the status code. Please see Status Codes and Troubleshooting for detailed information. |
Input Object Dimensions to Mech-Vision
This function dynamically inputs object dimensions into the Mech-Vision project. This function must be called BEFORE “mm_start_vis”.
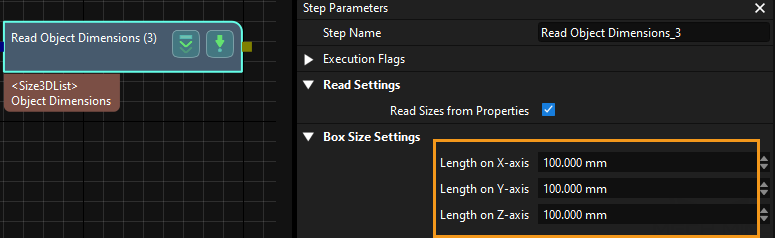
Get DO Signal List
This function obtains the planned DO Signal list for controlling multiple sections of a sectioned vacuum gripper. “mm_get_vizdata” must be called BEFORE this function.
Please configure the Mech-Viz project based on the example project in XXXX/Mech-Center-xxx/tool/viz_project/suction_zone , and set the suction cup configuration file in the Mech-Viz project.
|
Input TCP to Mech-Viz
This function dynamically inputs a TCP pose to the External Move Step in Mech-Viz. Please use this function based on the example project in XXXX/Mech-Center-xxx/tool/viz_project/outer_move
, and place the External Move Step at a proper position in the workflow.
“mm_start_viz” must be called BEFORE this function.
Calibration
This function is used for hand-eye calibration (camera extrinsic parameter calibration). It automates the calibration process in conjunction with the Camera Calibration function in Mech-Vision. For detailed instructions, see Kawasaki Calibration Process.
Get Custom Output Data from Mech-Vision
This function obtains the custom output data from the corresponding Mech-Vision project. “Custom output data” refers to the data output by ports other than poses and labels of the “Procedure Out” Step. The output ports can be customized if the “Port Type” parameter of the Step is set to “Custom”. The custom port outputs are sorted in alphabetical order of the custom port names.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received vision points. |
.ret |
Name of the variable for storing the received status code. |
After this function is called, the poses and corresponding labels of returned vision points can be read and stored using the function “mm_get_pose(.index,.&targetpos,.label,.speed)”. The custom output data is stored in two-dimensional array “vis_custom_data[]”.
Get Waypoint from Mech-Viz
This function is used for obtaining the planned path from Mech-Viz. The waypoint may be for a Vision Move Step, or for one of the other move-type Steps. A waypoint may contain pose, velocity, gripper info, object info, etc.
Waypoints in a planned path can be divided into the following three types.
-
Normal motion waypoints (not including the Vision Move waypoint): contain information such as motion type (joint motion or linear motion), tool number, and velocity.
-
Vision Move waypoints, containing information such as label, number of workobjects that have been picked, number of workobjects picked this time, edge/corner ID of the suction cup, the TCP offset, workobject orientation, and workobject dimensions.
-
Vision Move waypoints including custom data. In this case, the port type of the “Procedure Out” Step in the Mech-Vision project should be “Custom”.
Parameter
Input parameter | Description |
---|---|
.getpos_type |
Expected format of the returned data, see the following table for explanations. |
Below are the explanations of the four possible data formats that can be returned by this function.
Value of the .getpos_type parameter | Format of returned data (Explained below) |
---|---|
1 |
Pose (joint positions), motion type, tool number, velocity, number of custom ports, custom port output 1, custom port output 2, … custom port output N |
2 |
Pose (TCP), motion type, tool number, velocity, number of custom ports, custom port output 1, custom port output 2, … custom port output N |
3 |
Pose (joint positions), motion type, tool number, velocity, depalletizing planning data, number of custom ports, custom port output 1, custom port output 2, … custom port output N |
4 |
Pose (TCP), motion type, tool number, velocity, depalletizing planning data, number of custom ports, custom port output 1, custom port output 2, … custom port output N |
Pose
The pose of the waypoint can be in the form of joint positions (in degree) or TCP, which is composed of Cartesian coordinates (XYZ in mm) and Euler angles (ABC in degree).
Motion type
-
1
: joint motion, MOVEJ -
2
: linear motion, MOVEL
Tool number
The index number of the tool to be used at this waypoint. -1 indicates no tool is used.
Velocity
Velocity percentage of the waypoint. It is determined by multiplying the velocity setting specified in the corresponding move-type step by the global velocity setting in Mech-Viz. The result is expressed as a percentage.
Depalletizing planning data
Data used in planning multi-pick depalletizing tasks. This data is part of the waypoint of the “Vision Move” Step. The following data are included:
-
Label: composed of 10 integers, if fewer than 10 labels are obtained, the rest of the digits are filled with 0.
-
Number of picked workobjects.
-
Number of workobjects to be picked this time.
-
Edge-corner ID of vacuum gripper: used to specify the gripper corner to which the workobject is aligned. It can be checked by double-clicking the corresponding tool in the Resources panel in Mech-Viz and then selecting Configure control logic.
-
TCP offset: The offset between the tool pose at the center of the to-pick workobjects and the actual tool pose.
-
Workobject orientation: whether the X-axis of the workobject reference frame is aligned with the X-axis of the tool reference frame (value: 0 or 1).
-
Dimensions of the workobjects combined.
Number of custom ports
The number of output ports excluding poses and labels when the “Port Type” parameter of the “Procedure Out” Step in the Mech-Vision project is set to “Custom”.
Custom port outputs
The outputs that exclude poses and labels when the “Port Type” parameter of the “Procedure Out” Step in the Mech-Vision project is set to “Custom”. These data are part of the waypoint of the “Vision Move” Step. The custom port outputs are sorted in alphabetical order of the custom port names.
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received waypoints. |
.vispos_num |
Name of the variable for storing the position of the first “Vision Move” waypoint in the path. For example, if the workflow is: Fixed-Point Move 1 → Fixed-Point Move 2 → Vision Move → Fixed-Point Move 3, the value of VisPose_Num, i.e., the position of the Vision Move Step, is 3. If there is no Vision Move Step in the workflow, the parameter value is 0. |
.ret |
Name of the variable for storing the received status code. |
If this function is called successfully, returned data will be saved in variables as shown below.
Data | Variable |
---|---|
Joint positions J1-J6 |
vis_pos_j1[], vis_pos_j2[], vis_pos_j3[], vis_pos_j4[], vis_pos_j5[], vis_pos_j6[] |
TCP pose (XYZOAT) |
vis_pos_x[], vis_pos_y[], vis_pos_z[], vis_pos_o[], vis_pos_a[], vis_pos_t[] |
Velocity |
vis_pos_spd[i] |
Motion type |
vis_pos_type[i] |
Tool number |
vis_pos_tool[i] |
Mech-Vision custom port outputs |
vis_custom_data[i, j] |
Depalletizing planning data |
vis_plan_result[i, j] |
Example
CALL mm_get_plandata(3,pos_num,vis_index,statuscode)
This example obtains the planned path that includes custom port outputs from Mech-Viz, and the value of .getpos_type is 3. The number of waypoints received is stored in pos_num. The position of the Vision Move waypoint is stored in vis_index. The status code is stored in statuscode.
Get Result of Step “Path Planning” in Mech-Vision
This function obtains the collision-free path planned by the “Path Planning” Step from the corresponding Mech-Vision project.
The Port Type parameter of the “Procedure Out” Step in the Mech-Vision project must be set to “Predefined (robot path)”.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
.getpos_type |
The pose type of waypoints returned by the “Path Planning” Step.
|
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received waypoints. |
.vispos_num |
Name of the variable for storing the position of the “Vision Move” waypoint in the entire planned path in the Path Planning tool. For example, if the path is composed of Steps Fixed-Point Move_1, Fixed-Point Move_2, Vision Move, and Fixed-Point Move_3 sequentially, the position of Vision Move is 3. If the path does not contain any Vision Move waypoint, the return value is 0. |
.ret |
Name of the variable for storing the received status code. |
Example
CALL mm_get_vispath(1,1,posnum,vis_index,statuscode)
This example obtains the planned path from Mech-Vision project whose ID is 1 in the form of joint positions.The number of waypoints received is stored in posnum, the position of the Vision Move waypoint is stored in vis_index, and the status code is stored in statuscode.