Kawasaki Standard Interface Functions
When writing your own program, pay attention to the following:
-
Parameters in a function should be separated by commas.
-
Variable-type parameters should use local variables, which take effect only in the program.
-
Input or Output parameters can be customized for functions.
-
Arguments in the program: the input arguments can be constants, global variables or local variables, and the output arguments can be global variables or local variables.
Initialize Communication
This function sets the host IP address, port number, and wait time for TCP/IP communication. The parameters set in this function are global variables. Therefor, the robot program only needs to call this function once.
Start Mech-Vision Project
This function is used for applications that use Mech-Vision. It runs the corresponding project to acquire and process data. It applies for applications that use Mech-Vision but not Mech-Viz.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
.pos_num_need |
The expected number of vision points for Mech-Vision to send. Value range: 0 to 20, in which 0 indicates that all vision points will be sent. |
.sendpos_type |
The type of the robot pose to be sent to Mech-Vision. Value range: 0 to 3.
|
.#start_vis |
The robot joint positions of the start point when .sendpos_type is set to 3. When .sendpos_type is set other values, this parameter can be directly set to #start_vis. |
Example
-
Example 1:
CALL mm_start_vis(1,1,1,#start_vis)
This example triggers the Mech-Vision project whose ID is 1 to run; the Mech-Vision project is expected to send back one vision point; the robot sends its current joint positions and flange pose as the image-capturing pose to Mech-Vision. The #start_vis parameter is not specified.
-
Example 2:
CALL mm_start_vis(1,1,3,#start_vis)
This example triggers the Mech-Vision project whose ID is 1 to run; the Mech-Vision project is expected to send back one vision point; The robot sends the joint positions stored in the #start_vis variable to Mech-Vision as the start point of the planned path for the simulated robot in the Path Planning tool.
Get Vision Target(s)
This function is used in scenarios where only Mech-Vision is used, but Mech-Viz is not. After Mech-Vision is triggered, this function can be used to obtain results from the Vision Look Step.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of returned poses. |
.ret |
Name of the variable for storing the status code. Please see Status Codes and Troubleshooting for detailed information. |
Start Mech-Viz Project
This function is used in scenarios where both the Mech-Vision project and the Mech-Viz project are used. This function starts the Mech-Viz project and the corresponding Mech-Vision project, and sets the initial pose of the simulated robot in Mech-Viz.
Parameter
Input parameter | Description |
---|---|
.sendpos_type |
Robot pose type. Value range: 0 to 2.
|
.#start_viz |
The robot joint positions of the start point when .sendpos_type is set to 2. When .sendpos_type is set other values, this parameter can be directly set to #start_viz. |
Example
-
Example 1:
CALL mm_start_viz(1,#start_viz)
This function triggers the Mech-Viz project to run and sends the current joint positions and flange pose of the robot to Mech-Viz.
-
Example 2:
CALL mm_start_viz(2,#start_viz)
This function triggers the Mech-Viz project to run and sends the joint positions stored in #start_viz to Mech-Viz.
Get Planned Path from Mech-Viz
This function obtains the path planned by a Mech-Viz project.
Parameter
Input parameter | Description |
---|---|
.getpos_type |
Pose type of the waypoints on the planned path. Value range: 1 or 2.
|
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received poses. |
.vispos_num |
Name of the variable for storing the position of the first Vision Move waypoint in the path. For example, if the path is: Fixed-Point Move 1 → Fixed-Point Move 2 → Vision Move → Fixed-Point Move 3, the value of VisPose_Num (the position of the Vision Move Step) is 3. If there is no Vision Move Step in the path, the parameter value is 0. |
.ret |
Name of the variable for storing the status code. Please see Status Codes and Troubleshooting for detailed information. |
Example
CALL mm_get_vizdata(2,posnum,vis_index,statuscode)
This example obtains the planned path from Mech-Viz in the form of TCPs. The number of received poses is stored in variable posnum, the position of the Vision Move waypoint is stored in variable vis_index, and the received status code is stored in variable statuscode.
Obtain Vision Results
This function stores the TCP pose of a vision point returned by Mech-Vision or a waypoint returned by Mech-Viz in the specified variable.
Parameter
Input parameter | Description |
---|---|
.index |
The index of the vision point or waypoint whose TCP pose will be stored. |
Output parameter | Description |
---|---|
.&targetpos |
The name of the pose variable for storing the specified TCP pose. The “&” needs to be put in front of the parameter to indicate that the value of this variable is a TCP pose. |
.label |
Name of the variable for storing the label corresponding to the specified TCP pose. |
.speed |
Name of the variable for storing the velocity corresponding to the specified TCP pose. |
Store the Joint Positions of a Vision Point Obtained from Mech-Viz
This function stores the joint positions of a waypoint on the planned path returned by Mech-Viz in the specified variable.
Parameter
Input parameter | Description |
---|---|
.index |
The index of the vision point or waypoint whose TCP pose will be stored. |
Output parameter | Description |
---|---|
.#targetpos |
Name of the JPs variable for storing a specified set of joint positions. The “#” needs to be put in front of the parameter to indicate that the value of this variable is a set of joint positions. |
.label |
Name of the variable for storing the label corresponding to the specified TCP pose. |
.speed |
Name of the variable for storing the velocity corresponding to the specified TCP pose. |
Switch Mech-Vision Recipe
This function specifies which parameter recipe of the Mech-Vision project to use. This function must be called BEFORE “mm_start_vis”.
Select Mech-Viz Branch
This function selects along which branch the Mech-Viz project should proceed. Such branching is achieved by adding “Branch by Msg” Step(s) to the Mech-Viz project. This function specifies which exit port such Step(s) should take. “mm_start_viz” must be called BEFORE this function. When the Mech-Viz project executes to the “Branch by Msg” Step, it will wait for “mm_set_branch” to send the exit port of the branch.
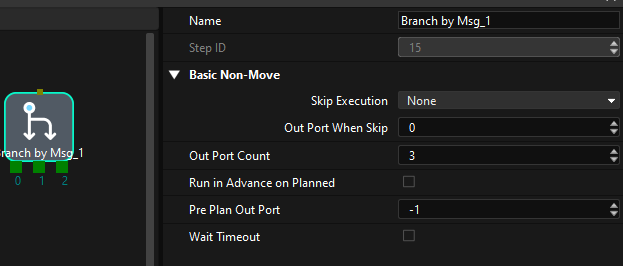
Parameter
Input parameter | Description |
---|---|
.branch_num |
Step ID of the “Branch by Msg” Step. Its value is an integer. The Step ID is displayed in the parameters of the Step. |
.exit_num |
The number of the exit port to take plus 1. For example, to select exit port 0 in Mech-Viz, set the value of this parameter to 1. Value range: 1 to 99. |
Set Move Index
This function sets the value for the Current Index parameter of a mote-type Step. Move-type Steps include Move by List, Move by Grid, Custom Pallet Pattern, and Predefined Pallet Pattern. “mm_start_viz” must be called BEFORE this function.
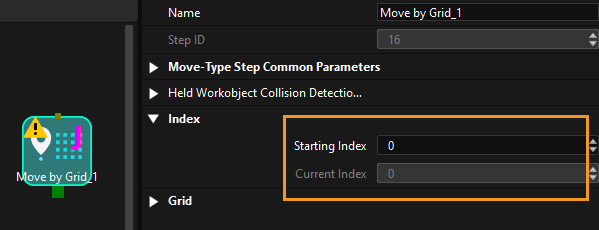
Parameter
Input parameter | Description |
---|---|
.skill_num |
ID of the Step, an integer. The Step ID is displayed in the parameters of the Step. |
.index_num |
The index value that should be set the next time this Step is executed. When this function is sent, the current index value in Mech-Viz will become the parameter value minus 1. When the Mech-Viz project runs to the Step specified by this subprogram, the Current Index value in Mech-Viz will be increased by 1 to become the parameter’s value. |
Get Software Status
This function is used to obtain the software execution status of Mech-Vision, Mech-Viz, and Mech-Center. Currently, this function can only be used to check whether Mech-Vision is ready to run.
Parameter
Output parameter | Description |
---|---|
.ret |
Name of the variable for storing the status code. Please see Status Codes and Troubleshooting for detailed information. |
Input Object Dimensions to Mech-Vision
This function dynamically inputs object dimensions into the Mech-Vision project. This function must be called BEFORE “mm_start_vis”.
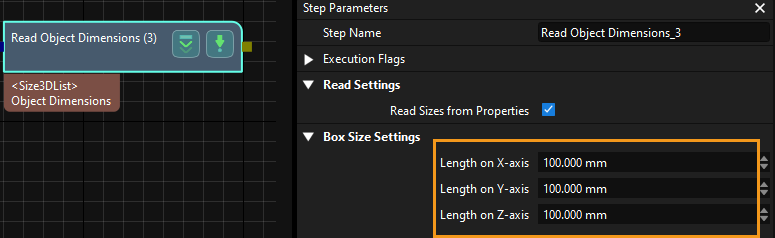
Read Mech-Viz Step Parameter
This function reads the parameter value of the specified Step in the Mech-Viz project.
Parameter
Input parameter | Description |
---|---|
.group_num |
This parameter specifies the Config ID field defined in the property_config file. |
From the toolbar of Mech-Vision, go to . Click Property Configuration to open the property_config file.![]() |
Before calling this function, users should define a Config ID and its corresponding Step ID and parameter key name in the following format in the property_config file.
read, Config ID, Step ID, parameter key name
read |
Indicates that this line is used to read the parameter value of a Step. |
Config ID |
Specifies a unique ID, which in a positive integer. One Config ID corresponds to only one parameter value of a Step. To read multiple parameter values, you should set different Config IDs. |
Step ID |
The Step ID of the Step whose parameter is to be read. |
Parameter key name |
Specifies the key name of the parameter whose value the robot requires to read. |
|
The property_config file can have multiple read commands. The Config ID in these commands must be different. |
Output parameter | Description |
---|---|
.return_value |
The value of the parameter of the specified Step. |
Example
In the line below, “5” is the Config ID; “3” is the Step ID; and “xCount” is the parameter key name. Add this line to the property_config file. Add this line to the property_config file.
read, 5, 3, xCount
When the robot calls the function below, it receives the value of the parameter whose key name is “xCount”.
CALL mm_get_property(5,param_value)
In this example, the value of the parameter corresponding to xCount in Step 3 of the Mech-Viz project is stored in the param_value variable.
Set Mech-Viz Step Parameter
This function sets the parameter value of the specified Step in the Mech-Viz project.
Parameter
Input parameter | Description |
---|---|
.group_num |
This parameter specifies the Config ID field defined in the property_config file. |
From the toolbar of Mech-Vision, go to . Click Property Configuration to open the property_config file.![]() |
Before sending this command, users should define a Config ID and its corresponding Step ID, parameter key name and parameter value in the following format in the property_config file.
write, Config ID, Step ID, parameter key name, parameter value
write |
Indicates that this line is used to set the parameter value of a Step. |
Config ID |
Specifies an ID, which is a positive integer and can be used repeatedly. |
Step ID |
Specifies the Step whose parameter value the robot requires to read. |
Parameter key name |
Specifies the key name of the parameter whose value the robot requires to set. |
Parameter value |
Specifies the value that the robot sets for the parameter. |
|
Example
In the line below, “1” is the Config ID, and “3” is the Step ID. “xOffset”, “yOffset” and “zOffset” are parameter key names; and “0.000000”, “1.000000” and “2.000030” are their respective values. Add this line to the property_config file.
write, 1, 3, xOffset, 0.000000
write, 1, 3, yOffset, 1.000000
write, 1, 3, zOffset, 2.000030
When the robot calls the function below, Mech-Viz sets the values of the parameters whose key names are “xOffset”, “yOffset” and “zOffset” respectively to “0.000000”, “1.000000” and “2.000030”.
CALL mm_set_property(1)
In this example, the values of the parameters whose key names are “xOffset”, “yOffset” and “zOffset” are respectively set to “0.000000”, “1.000000”, and “2.000030”.
Get Gripper DO List
This function obtains the gripper DO list planned by Mech-Vision or Mech-Viz. To apply the DO signals to the gripper, call the mm_set_dolist function.
|
Set Gripper DO List
This function sets the obtained DO signals to the robot’s gripper.
mm_get_dolist must be called BEFORE this function. |
Example
CALL mm_get_vizdata(2,posnum,vis_index,statuscode) ;receive path
CALL mm_get_dolist(0) ;receive DO from viz
CALL mm_get_pose(vis_index,pt1,label1,speed1) ; save the pick point to pt1
LAPPRO pt1,200
LMOVE pt1 ;move to pick point
CALL mm_set_dolist ;turn on DO for picking
twait 1 ;wait for gripping
LDEPART 200
In this example, after the robot moves to the Vision Move waypoint, the program calls mm_set_dolist to set the specific DO signals of the gripper to ON.
Calibration
This function is used for hand-eye calibration (camera extrinsic parameter calibration). It automates the calibration process in conjunction with the Camera Calibration function in Mech-Vision. For detailed instructions, see Kawasaki Calibration Process.
Get Custom Output Data from Mech-Vision
This function obtains the custom output data from the corresponding Mech-Vision project. “Custom output data” refers to the data output by ports other than poses and labels of the “Procedure Out” Step. The output ports can be customized if the “Port Type” parameter of the Step is set to “Custom”. The custom port outputs are sorted in alphabetical order of the custom port names.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received vision points. |
.count |
Name of the variable for storing the number of elements received from custom port. |
.ret |
Name of the variable for storing the received status code. |
After this function is called, the poses and corresponding labels of returned vision points can be read and stored using the function “mm_get_pose(.index,.&targetpos,.label,.speed)”. The custom output data is stored in two-dimensional array “vis_custom_data[]”.
Example
CALL mm_get_dy_data(1,pos_num,count,statuscode)
This example obtains the vision result that includes custom port outputs from Mech-Vision project No.1. The number of vision points received is stored in pos_num, the number of elements in custom outputs is stored in count, and the status code is stored in statuscode.
Get Vision Move Data or Custom Output Data
This function obtains Vision Move output from the Mech-Vision project, or the Vision Move data or custom output data from the Mech-Viz project.
Parameter
Input parameter | Description |
---|---|
.resource |
This parameter specifies the source of the Vision Move output.
|
.getpos_type |
This parameter specifies the returned data format. Details are provided below. |
-
When the value of .resource is 0, the valid value range of .getpos_type is 1 to 4. Details are provided below.
Value of .getpos_type Format of returned data (Explained below) 1
Pose (joint positions), motion type, tool number, velocity, number of elements in custom output data, element 1 in custom output data, ... element N in custom output data
2
Pose (TCP), motion type, tool number, velocity, number of elements in custom output data, element 1 in custom output data, ... element N in custom output data
3
Pose (joint positions), motion type, tool number, velocity, Mech-Viz Vision Move data, depalletizing planning data, number of elements in custom output data, element 1 in custom output data, ... element N in custom output data
4
Pose (TCP), motion type, tool number, velocity, Mech-Viz Vision Move data, number of elements in custom output data, element 1 in custom output data, ... element N in custom output data
-
When the value of .resource is a positive integer, the valid value range of .getpos_type is 1 to 2. Details are provided below.
Value of .getpos_type Format of returned data (Explained below) 1
Pose (joint positions), motion type, tool ID, velocity, Mech-Vision Vision Move data
2
Pose (TCP), motion type, tool ID, velocity, Mech-Vision Vision Move data
Pose
The pose of the waypoint can be in the form of joint positions (in degree) or TCP, which is composed of Cartesian coordinates (XYZ in mm) and Euler angles (ABC in degree).
Motion type
-
1: Joint motion (MOVEJ)
-
2: Linear motion (MOVEL)
Tool ID
The ID of the tool to be used at this waypoint. The value “-1” means that no tool will be used at this waypoint.
Velocity
The velocity at this waypoint.
Vision Move data
Data output by the “Vision Move” Step in Mech-Vision or Mech-Viz, including labels of picked workobjects, number of picked workobjects, number of workobjects to be picked this time, edge/corner ID of vacuum gripper, TCP offset, orientation of workobject group, orientation of workobject, dimensions of workobject group.
Number of elements in custom output data
The number of elements in custom output data.
Element in custom output data
“Custom output data” refers to the data output by ports other than poses and labels of the “Procedure Out” Step in the Mech-Vision project. The output ports can be customized if the “Port Type” parameter of the Step is set to “Custom”. The custom port outputs are sorted in alphabetical order of the custom port names.
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received waypoints. |
.vispos_index |
Name of the variable for storing the position of the first “Vision Move” waypoint in the path. For example, if the path is: Fixed-Point Move 1 → Fixed-Point Move 2 → Vision Move 1 → Fixed-Point Move 3, the value of VisPose_Num, i.e., the position of the Vision Move Step, is 3. If there is no Vision Move Step in the path, the parameter value is 0. |
.ret |
Name of the variable for storing the received status code. |
If this function is called successfully, returned data will be saved in variables as shown below.
Data | Variable (both “i” and “j” starts from 1) |
---|---|
Joint positions (J1 - J6) |
vis_pos_j1[], vis_pos_j2[], vis_pos_j3[], vis_pos_j4[], vis_pos_j5[], vis_pos_j6[] |
TCP pose (XYZOAT) |
vis_pos_x[], vis_pos_y[], vis_pos_z[], vis_pos_o[], vis_pos_a[], vis_pos_t[] |
Velocity |
vis_pos_spd[i] |
Motion type |
vis_pos_type[i] |
Tool ID |
vis_pos_tool[i] |
Vision Move waypoint or not |
If vis_pos_vismove[i] is 1, the waypoint is a Vision Move waypoint. Otherwise, it is a non-Vision Move waypoint. |
Mech-Vision custom port outputs |
vis_custom_data[i,j]
|
Vision Move data of Mech-Vision or Mech-Viz |
vis_plan_result[i,j] Only when vis_pos_vismove[i] is 1, vis_custom_data[i,j] has a value. |
Content of the vis_plan_result[i,j] variable is explained in the table below.
Data | Description | Variable |
---|---|---|
Labels of picked workobjects |
Consists of 10 integers. By default, its value is ten 0s. |
vis_plan_result[i,1] ~ vis_plan_result[i.10] |
Number of picked workobjects |
The total number of workobjects that have been picked. |
vis_plan_result[i,11] |
Number of workobjects to be picked this time |
Number of workobjects to be picked this time |
vis_plan_result[i,12] |
Vacuum gripper edge/corner ID |
The ID of the edge/corner used to pick workobjects this time. |
vis_plan_result[i,13] |
TCP offset |
The XYZ offset between the center of the workobject group and the tool pose center. |
vis_plan_result[i,14] ~ vis_plan_result[i,16] |
Orientation of workobject group |
The relative position between the workobject group and the length of the vacuum gripper. The value is 0 or 1, where 0 stands for parallel and 1 for vertical. |
vis_plan_result[i,17] |
Orientation of workobject |
The relative position between the length of a workobject and that of the vacuum gripper. The value is 0 or 1, where 0 stands for parallel and 1 for vertical. |
vis_plan_result[i,18] |
Dimensions of workobject group |
The length, width and height of the workobject group to be picked this time. |
vis_plan_result[i,19] ~ vis_plan_result[i,21] |
Example
CALL mm_get_plandata(0,3,pos_num,vispos_index,statuscode)
This example obtains the planned path from Mech-Viz, and the value of .getpos_type is 3. The number of waypoints received is stored in pos_num. The position of the Vision Move waypoint is stored in vispos_index. The status code is stored in statuscode.
Get Result of Step “Path Planning” in Mech-Vision
This procedure obtains the collision-free path planned by the “Path Planning” Step from the corresponding Mech-Vision project.
The Port Type parameter of the “Procedure Out” Step in the Mech-Vision project must be set to “Predefined (robot path)”.
Parameter
Input parameter | Description |
---|---|
.job |
Mech-Vision project ID, which can be viewed before the project name in the Project List panel in Mech-Vision. |
.getpos_type |
The pose type of waypoints returned by the “Path Planning” Step.
|
Output parameter | Description |
---|---|
.pos_num |
Name of the variable for storing the number of received waypoints. |
.vispos_num |
Name of the variable for storing the position of the “Vision Move” waypoint in the entire planned path in the Path Planning tool. For example, if the path is composed of Steps Fixed-Point Move_1, Fixed-Point Move_2, Vision Move, and Fixed-Point Move_3 sequentially, the position of Vision Move is 3. If the path does not contain any Vision Move waypoint, the return value is 0. |
.ret |
Name of the variable for storing the received status code. |
Example
CALL mm_get_vispath(1,1,posnum,vis_index,statuscode)
This example obtains the planned path from Mech-Vision project No.1. Waypoints are obtained in the form of joint positions.The number of waypoints is stored in posnum, the position of the Vision Move waypoint is stored in vis_index, and the status code is stored in statuscode.