Example Program 12: MM_S12_Viz_ForLoop
Program Introduction
Description |
The robot triggers the Mech-Viz project to run, obtains the planned path, and then stores the planned path by looping for picking and placing. |
||
File path |
You can navigate to the installation directory of Mech-Vision and Mech-Viz and find the file by using the
|
||
Project |
Mech-Vision and Mech-Viz projects |
||
Prerequisites |
|
This example program is provided for reference only. Before using the program, please modify the program according to the actual scenario. |
Program Description
This part describes the MM_S12_Viz_ForLoop example program.
The MM_S12_Viz_ForLoop example program is similar to the MM_S2_Viz_Basic example program, except that MM_S2_Viz_Basic stores waypoints one by one and MM_S12_Viz_ForLoop stores waypoints by looping (the code of this feature is bolded). As such, only the feature of storing waypoints by looping is described in the following section. For information about the parts of MM_S12_Viz_ForLoop that are consistent with those of MM_S2_Viz_Basic, see Example Program 2: MM_S2_Viz_Basic. |
MODULE MM_S12_Viz_ForLoop
!----------------------------------------------------------
! FUNCTION: trigger Mech-Viz project and get planned path,
! get poses using for-loop structure
! Mech-Mind, 2023-12-25
!----------------------------------------------------------
!define local num variables
LOCAL VAR num pose_num:=0;
LOCAL VAR num status:=0;
LOCAL VAR num toolid{5}:=[0,0,0,0,0];
LOCAL VAR num vis_pose_num:=0;
LOCAL VAR num count:=0;
LOCAL VAR num label{5}:=[0,0,0,0,0];
!define local joint&pose variables
LOCAL CONST jointtarget home:=[[0,0,0,0,90,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]];
LOCAL CONST jointtarget snap_jps:=[[0,0,0,0,90,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]];
LOCAL PERS robtarget camera_capture:=[[302.00,0.00,558.00],[0,0,-1,0],[0,0,0,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]];
LOCAL PERS robtarget drop_waypoint:=[[302.00,0.00,558.00],[0,0,-1,0],[0,0,0,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]];
LOCAL PERS robtarget drop:=[[302.00,0.00,558.00],[0,0,-1,0],[0,0,0,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]];
LOCAL PERS jointtarget jps{5}:=
[
[[0,0,0,0,89,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]],
[[11.1329,49.0771,-36.9666,0.5343,79.2476,-169.477],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]],
[[11.2355,52.1281,-23.3996,0.5938,62.6295,-169.548],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]],
[[11.1329,49.0771,-36.9666,0.5343,79.2476,-169.477],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]],
[[0,0,0,0,90,0],[9E+9,9E+9,9E+9,9E+9,9E+9,9E+9]]
];
!define local tooldata variables
LOCAL PERS tooldata gripper1:=[TRUE,[[0,0,0],[1,0,0,0]],[0.001,[0,0,0.001],[1,0,0,0],0,0,0]];
PROC Sample_12()
!set the acceleration parameters
AccSet 50, 50;
!set the velocity parameters
VelSet 50, 1000;
!move to robot home position
MoveAbsJ home\NoEOffs,v3000,fine,gripper1;
!initialize communication parameters (initialization is required only once)
MM_Init_Socket "127.0.0.1",50000,300;
!move to image-capturing position
MoveL camera_capture,v1000,fine,gripper1;
!open socket connection
MM_Open_Socket;
!trigger Mech-Viz project
MM_Start_Viz 2,snap_jps;
!get planned path, 1st argument (1) means getting pose in JPs
MM_Get_VizData 1, pose_num, vis_pose_num, status;
!check whether planned path has been got from Mech-Viz successfully
IF status <> 2100 THEN
!add error handling logic here according to different error codes
!e.g.: status=2038 means no point cloud in ROI
Stop;
ENDIF
!close socket connection
MM_Close_Socket;
!save waypoints of planned path to local variables using for-loop structure
FOR i FROM 1 TO pose_num DO
count:=i;
MM_Get_Jps count,jps{count},label{count},toolid{count};
ENDFOR
!follow the planned path to pick, in this example waypoint 2 (jps{2}) is picking waypoint
!move to approach waypoint of picking
MoveAbsJ jps{1},v1000,fine,gripper1;
!move to picking waypoint
MoveAbsJ jps{2},v300,fine,gripper1;
!add object grasping logic here, such as "setdo DO_1, 1;"
Stop;
!move to departure waypoint of picking
MoveAbsJ jps{3},v1000,fine,gripper1;
!move to intermediate waypoint of placing
MoveJ drop_waypoint,v1000,z50,gripper1;
!move to approach waypoint of placing
MoveL RelTool(drop,0,0,-100),v1000,fine,gripper1;
!move to placing waypoint
MoveL drop,v300,fine,gripper1;
!add object releasing logic here, such as "setdo DO_1, 0;"
Stop;
!move to departure waypoint of placing
MoveL RelTool(drop,0,0,-100),v1000,fine,gripper1;
!move back to robot home position
MoveAbsJ home\NoEOffs,v3000,fine,gripper1;
ENDPROC
ENDMODULE
The workflow corresponding to the above example program code is shown in the figure below.
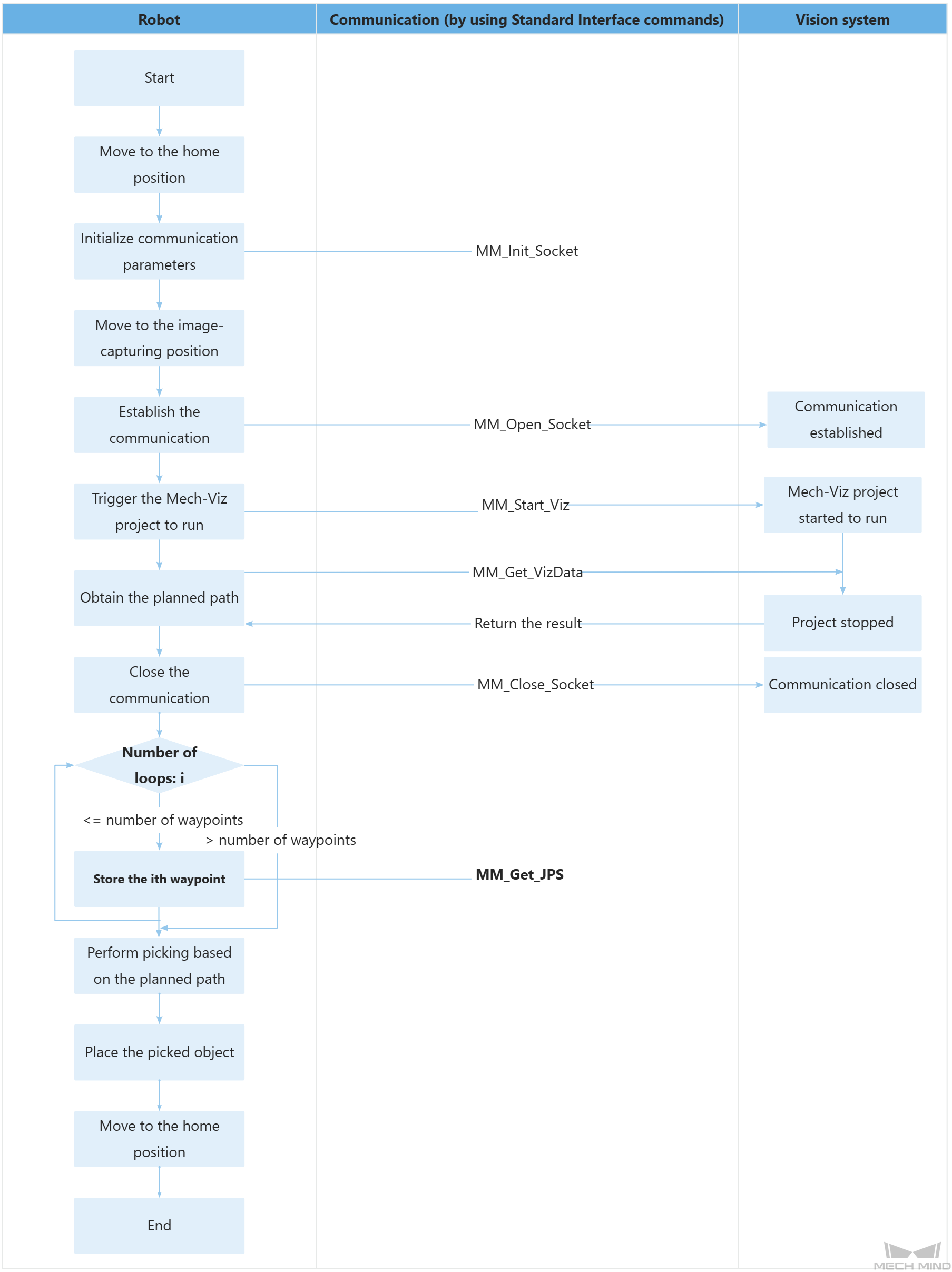
The table below describes the feature of storing the planed path in waypoints by looping. You can click the hyperlink to the command name to view its detailed description.
Feature | Code and description |
---|---|
Store the planned path by looping |
Assuming that the path planned in this example program contains three waypoints, the above for loop is equivalent to the following three commands in the MM_S2_Viz_Basic example program:
|