Operation Workflow
This topic introduces the basic workflow of using Mech-Eye API to control a laser profiler. The figure and descriptions in this topic use the class and method names of C++ Mech-Eye API. For the corresponding names of different languages, please refer to the example codes.
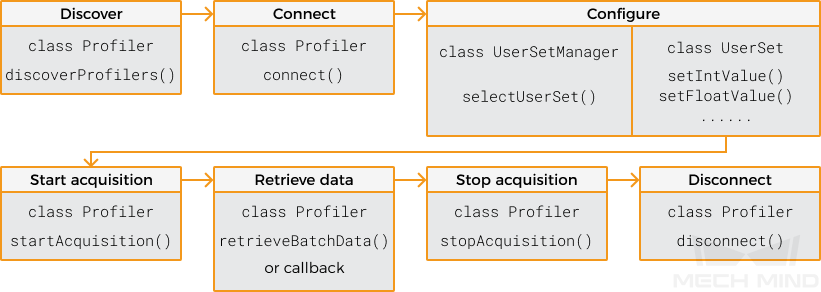
Discover
Call the discoverProfilers()
method in the Profiler
class to enumerate all currently connectable laser profilers and obtain the information of each laser profiler.
-
C++
-
C#
std::vector<mmind::eye::ProfilerInfo> profilerInfoList = mmind::eye::Profiler::discoverProfilers();
var profilerInfoList = Profiler.DiscoverProfilers();
Connect
After instantiating the Profiler
class, call the connect()
method in this class to connect to the corresponding laser profiler using the IP address or the device information obtained by the discoverProfilers()
method.
-
C++
-
C#
mmind::eye::Profiler profiler;
mmind::eye::ErrorStatus status = profiler.connect(profilerInfoList[inputIndex]);
mmind::eye::Profiler profiler;
mmind::eye::ErrorStatus status = profiler.connect("192.168.0.10");
var profiler = new Profiler();
var status = profiler.Connect(profilerInfoList[inputIndex]);
var profiler = new Profiler();
var status = profiler.Connect("192.168.0.10");
Configure
Call the methods in the UserSetManager
and UserSet
classes to select the parameter group on the laser profiler and adjust the parameters.
-
Call the
selectUserSet
method in theUserSetManager
class to select the parameter group to be used.You can obtain all the available parameter groups on the laser profiler through the getAllUserSetNames()
method in this class.-
C++
-
C#
mmind::eye::UserSetManager userSetManager = profiler.userSetManager(); std::vector<std::string> userSets; auto status = userSetManager.getAllUserSetNames(userSets); status = userSetManager.selectUserSet(userSets.front());
var userSetManager = profiler.UserSetManager(); List<string> userSets = new List<string>(); var status = userSetManager.GetAllUserSetNames(ref userSets); status = userSetManager.SelectUserSet(userSets[0]);
-
-
Call the
getAvailableParameters()
method in theUserSet
class to obtain the information of all the parameters in the current parameter group and check the data type of each parameter.-
C++
-
C#
mmind::eye::UserSet currentUserSet = profiler.currentUserSet(); std::vector<Parameter*> parameters = currentUserSet.getAvailableParameters();
var currentUserSet = profiler.CurrentUserSet(); var parameters = currentUserSet.GetAvailableParameters();
-
-
Call the
getIntValue()
and other similar methods in theUserSet
class to obtain the current value of a parameter.-
C++
-
C#
int exposureTime = 0; auto status = currentUserSet.getIntValue(mmind::eye::brightness_settings::ExposureTime::name, exposureTime);
int exposureTime = 0; var status = currentUserSet.GetIntValue(MMind.Eye.BrightnessSettings.ExposureTime.Name, ref exposureTime);
-
-
Call the
setIntValue()
and other similar methods in theUserSet
class to set the value of a parameter.-
C++
-
C#
auto status = currentUserSet.setIntValue(mmind::eye::brightness_settings::ExposureTime::name, 20);
var status = currentUserSet.SetIntValue(MMind.Eye.BrightnessSettings.ExposureTime.Name, 20);
-
-
Call the
saveAllParametersToDevice()
method in theUserSet
class to save the set parameter values to the laser profiler.-
C++
-
C#
auto status = currentUserSet.SaveAllParametersToDevice();
var status = currentUserSet.SaveAllParametersToDevice();
-
Start Acquisition
Call the startAcquisition()
method in the Profiler
class to enter the laser profiler into the acquisition ready status, where it can accept trigger signals for scanning.
-
If you use externally input signals to trigger data acquisition, the laser profiler starts to scan when it receives such a signal.
-
C++
-
C#
const auto status = currentUserSet.setEnumValue( mmind::eye::trigger_settings::DataAcquisitionTriggerSource::name, static_cast<int>(mmind::eye::trigger_settings::DataAcquisitionTriggerSource::Value::External)); auto status = profiler.startAcquisition();
var status = currentUserSet.SetEnumValue( MMind.Eye.TriggerSettings.DataAcquisitionTriggerSource.Name, (int)MMind.Eye.TriggerSettings.DataAcquisitionTriggerSource.Value.External); var status = profiler.StartAcquisition();
-
-
If you use software to trigger data acquisition, call the
triggerSoftware()
method in theProfiler
class to trigger scanning.-
C++
-
C#
const auto status = currentUserSet.setEnumValue( mmind::eye::trigger_settings::DataAcquisitionTriggerSource::name, static_cast<int>(mmind::eye::trigger_settings::DataAcquisitionTriggerSource::Value::Software)); auto status = profiler.startAcquisition(); status = profiler.triggerSoftware();
var status = currentUserSet.SetEnumValue( MMind.Eye.TriggerSettings.DataAcquisitionTriggerSource.Name, (int)MMind.Eye.TriggerSettings.DataAcquisitionTriggerSource.Value.Software); var status = profiler.StartAcquisition(); status = profiler.TriggerSoftware();
-
Retrieve Data
The data obtained by the laser profiler in a single round of data acquisition is returned in several batches. All batches of data must be retrieved first before the intensity image and depth map can be generated.
You can retrieve the data from the laser profiler in two ways:
-
Call the
retrieveBatchData()
method in theProfiler
class. Calling this method once can obtain a single batch of data. -
Define a callback function and call the
registerAcquisitionCallBack()
method in theProfiler
class to register the callback function.The callback function must be defined and registered before the Profiler
class function is called.
The example codes for the two ways of retrieving data are provided below.
-
Call the
retrieveBatchData()
method in theProfiler
class:-
C++
-
C#
int dataWidth = 0; auto status = currentUserSet.getIntValue(mmind::eye::scan_settings::DataPointsPerProfile::name, dataWidth); mmind::eye::ProfileBatch batch(dataWidth); status = profiler.retrieveBatchData(batch);
int dataWidth = 0; var status = currentUserSet.GetIntValue(MMind.Eye.ScanSettings.DataPointsPerProfile.Name, ref dataWidth); var batch = new ProfileBatch((ulong)dataWidth); MMind.Eye.ErrorStatus status = profiler.RetrieveBatchData(ref batch);
-
-
Use a callback function:
-
C++
-
C#
namespace{ std::mutex kMutex; void callbackFunc(const mmind::eye::ProfileBatch& batch, void* pUser) { std::unique_lock<std::mutex> lock(kMutex); auto* outPutBatch = static_cast<mmind::eye::ProfileBatch*>(pUser); outPutBatch->append(batch); } } int dataPoints = 0; showError(currentUserSet.getIntValue(mmind::eye::scan_settings::DataPointsPerProfile::name, dataPoints)); mmind::eye::ProfileBatch profileBatch(dataPoints); profiler.registerAcquisitionCallback(callbackFunc, &profileBatch);
private static readonly Mutex mut = new Mutex(); private static void CallbackFunc(ref ProfileBatch batch, IntPtr pUser) { mut.WaitOne(); GCHandle handle = GCHandle.FromIntPtr(pUser); var outputBatch = (handle.Target as ProfileBatch); outputBatch.Append(batch); mut.ReleaseMutex(); } int dataPoints = 0; Utils.ShowError(currentUserSet.GetIntValue(MMind.Eye.ScanSettings.DataPointsPerProfile.Name, ref dataPoints)); var profileBatch = new ProfileBatch((ulong)dataPoints); GCHandle handle = GCHandle.Alloc(profileBatch); IntPtr param = (IntPtr)handle; profiler.RegisterAcquisitionCallback(CallbackFunc, param);
-
Stop Acquisition
Call the stopAcquisition()
method in the Profiler
class to stop the acquisition ready status of the laser profiler, in order to avoid accidental triggering of scanning.
-
C++
-
C#
profiler.stopAcquisition();
profiler.StopAcquisition();
Disconnect
Call the disconnect()
method in the Profiler
class to disconnect from the current laser profiler.
-
C++
-
C#
profiler.disconnect();
profiler.Disconnect();
This topic introduced the basic workflow of using Mech-Eye API to control a laser profiler. The next topic provides the reference manual for Mech-Eye API.